C++ program to add two polynomials.
C++ program to add two polynomials using array
#include<iostream>
using namespace std;
int addpoly(int (&a)[10][2],int (&b)[10][2],int (&c)[10][2],int n1,int n2)
{
int i=0,j=0,k=0;
while(1)
{
if(a[i][1]<b[j][1])
{
c[k][0]=b[j][0];
c[k][1]=b[j][1];
k++,j++;
}
else if(a[i][1]>b[j][1])
{
c[k][0]=a[i][0];
c[k][1]=a[i][1];
k++,i++;
}
else
{
c[k][0]=a[i][0]+b[j][0];
c[k][1]=a[i][1];
i++,j++,k++;
}
if(j==n2)
{
while(i<n1)
{
c[k][0]=a[i][0];
c[k][1]=a[i][1];
k++,i++;
}
}
if(i==n1)
{
while(j<n2)
{
c[k][0]=b[j][0];
c[k][1]=b[j][1];
k++,j++;
}
}
if(i==n1)
break;
}
return k;
}
void print(int (&d)[10][2],int n)
{
for(int i=0;i<n-1;i++)
cout<<d[i][0]<<"x^"<<d[i][1]<<" + ";
cout<<d[n-1][0]<<"x^"<<d[n-1][1]<<endl<<endl;
}
void getpoly(int (&d)[10][2],int &n)
{
cout<<"How many terms in polynomial ";
cin>>n;
cout<<"Enter coefficient and exponent in decreasing order"<<endl;
for(int i=0;i<n;i++)
cin>>d[i][0]>>d[i][1];
}
int main()
{
int a[10][2],b[10][2],c[10][2],n1,n2,n3;
getpoly(a,n1);
print(a,n1);
getpoly(b,n2);
print(b,n2);
n3=addpoly(a,b,c,n1,n2);
cout<<"Addition of two polynomials"<<endl;
print(c,n3);
return 0;
}
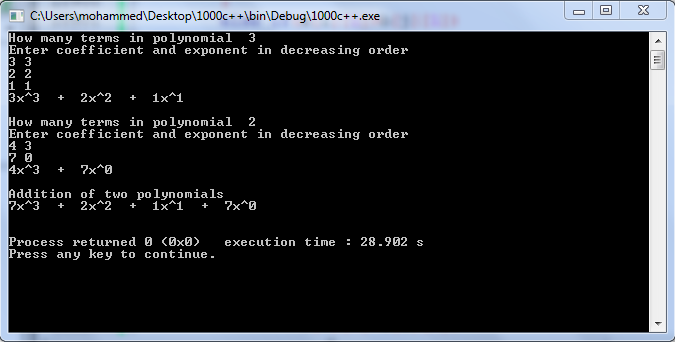
using namespace std;
int addpoly(int (&a)[10][2],int (&b)[10][2],int (&c)[10][2],int n1,int n2)
{
int i=0,j=0,k=0;
while(1)
{
if(a[i][1]<b[j][1])
{
c[k][0]=b[j][0];
c[k][1]=b[j][1];
k++,j++;
}
else if(a[i][1]>b[j][1])
{
c[k][0]=a[i][0];
c[k][1]=a[i][1];
k++,i++;
}
else
{
c[k][0]=a[i][0]+b[j][0];
c[k][1]=a[i][1];
i++,j++,k++;
}
if(j==n2)
{
while(i<n1)
{
c[k][0]=a[i][0];
c[k][1]=a[i][1];
k++,i++;
}
}
if(i==n1)
{
while(j<n2)
{
c[k][0]=b[j][0];
c[k][1]=b[j][1];
k++,j++;
}
}
if(i==n1)
break;
}
return k;
}
void print(int (&d)[10][2],int n)
{
for(int i=0;i<n-1;i++)
cout<<d[i][0]<<"x^"<<d[i][1]<<" + ";
cout<<d[n-1][0]<<"x^"<<d[n-1][1]<<endl<<endl;
}
void getpoly(int (&d)[10][2],int &n)
{
cout<<"How many terms in polynomial ";
cin>>n;
cout<<"Enter coefficient and exponent in decreasing order"<<endl;
for(int i=0;i<n;i++)
cin>>d[i][0]>>d[i][1];
}
int main()
{
int a[10][2],b[10][2],c[10][2],n1,n2,n3;
getpoly(a,n1);
print(a,n1);
getpoly(b,n2);
print(b,n2);
n3=addpoly(a,b,c,n1,n2);
cout<<"Addition of two polynomials"<<endl;
print(c,n3);
return 0;
}
OUTPUT
C++ program to add two polynomials using linked list
#include<iostream>
#include<conio.h>
using namespace std;
struct links
{
int exp,coef;
links *next;
};
class linkedlist
{
links *start1,*start2,*start3;
public:
void create(links **);
void add();
void display(links**);
links** getstart1()
{
return &start1;
}
links** getstart2()
{
return &start2;
}
links** getstart3()
{
return &start3;
}
};
void linkedlist::add()
{
links *temp3,*temp1,*temp2;
int flag=1;
temp1=start1;
temp2=start2;
while(temp1!=NULL)
{
if(temp1->exp>temp2->exp)
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
}
temp1=temp1->next;
}
else if(temp1->exp<temp2->exp)
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
}
temp2=temp2->next;
}
else
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef+temp2->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef+temp1->coef;
}
temp2=temp2->next;
temp1=temp1->next;
}
if(temp1==NULL)
{
while(temp2!=NULL)
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
temp2=temp2->next;
}
temp3->next=NULL;
}
if(temp2==NULL)
{
while(temp1!=NULL)
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
temp1=temp1->next;
}
temp3->next=NULL;
}
}
}
void linkedlist::create(links **start)
{
int exp,coef;
links *temp;
char ch;
cout<<"Enter the polynomial in descending order of exponent "<<endl;
cout<<"Enter the coefficient and Exponent of the term ";
cin>>coef>>exp;
temp=new links;
temp->coef=coef;
temp->exp=exp;
temp->next=NULL;
*start=temp;
cout<<"Want to add more terms (Y/N)"<<endl;
ch=getch();
while(ch!='n'&&ch!='N')
{
cout<<"Enter the coefficient and Exponent of the term ";
cin>>coef>>exp;
temp->next=new links;
temp=temp->next;
temp->coef=coef;
temp->exp=exp;
temp->next=NULL;
cout<<"Want to add more terms (Y/N)"<<endl;
ch=getch();
}
}
void linkedlist::display(links**start)
{
links *temp=*start;
while(temp->next!=NULL)
{
cout<<temp->coef<<"x^"<<temp->exp<<" + ";
temp=temp->next;
}
cout<<temp->coef<<"x^"<<temp->exp;
}
int main()
{
linkedlist addlist;
cout<<"Create First polynomial list "<<endl;
addlist.create(addlist.getstart1());
cout<<"Create a Second polynomial list "<<endl;
addlist.create(addlist.getstart2());
cout<<"\nFirst polynomial \n";
addlist.display(addlist.getstart1());
cout<<"\nSecond polynomial \n";
addlist.display(addlist.getstart2());
addlist.add();
cout<<"\nAddition of two polynomial \n";
addlist.display(addlist.getstart3());
return 0;
}
#include<conio.h>
using namespace std;
struct links
{
int exp,coef;
links *next;
};
class linkedlist
{
links *start1,*start2,*start3;
public:
void create(links **);
void add();
void display(links**);
links** getstart1()
{
return &start1;
}
links** getstart2()
{
return &start2;
}
links** getstart3()
{
return &start3;
}
};
void linkedlist::add()
{
links *temp3,*temp1,*temp2;
int flag=1;
temp1=start1;
temp2=start2;
while(temp1!=NULL)
{
if(temp1->exp>temp2->exp)
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
}
temp1=temp1->next;
}
else if(temp1->exp<temp2->exp)
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
}
temp2=temp2->next;
}
else
{
if(flag)
{
start3=new links;
temp3=start3;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef+temp2->coef;
flag=0;
}
else
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef+temp1->coef;
}
temp2=temp2->next;
temp1=temp1->next;
}
if(temp1==NULL)
{
while(temp2!=NULL)
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp2->exp;
temp3->coef=temp2->coef;
temp2=temp2->next;
}
temp3->next=NULL;
}
if(temp2==NULL)
{
while(temp1!=NULL)
{
temp3->next=new links;
temp3=temp3->next;
temp3->exp=temp1->exp;
temp3->coef=temp1->coef;
temp1=temp1->next;
}
temp3->next=NULL;
}
}
}
void linkedlist::create(links **start)
{
int exp,coef;
links *temp;
char ch;
cout<<"Enter the polynomial in descending order of exponent "<<endl;
cout<<"Enter the coefficient and Exponent of the term ";
cin>>coef>>exp;
temp=new links;
temp->coef=coef;
temp->exp=exp;
temp->next=NULL;
*start=temp;
cout<<"Want to add more terms (Y/N)"<<endl;
ch=getch();
while(ch!='n'&&ch!='N')
{
cout<<"Enter the coefficient and Exponent of the term ";
cin>>coef>>exp;
temp->next=new links;
temp=temp->next;
temp->coef=coef;
temp->exp=exp;
temp->next=NULL;
cout<<"Want to add more terms (Y/N)"<<endl;
ch=getch();
}
}
void linkedlist::display(links**start)
{
links *temp=*start;
while(temp->next!=NULL)
{
cout<<temp->coef<<"x^"<<temp->exp<<" + ";
temp=temp->next;
}
cout<<temp->coef<<"x^"<<temp->exp;
}
int main()
{
linkedlist addlist;
cout<<"Create First polynomial list "<<endl;
addlist.create(addlist.getstart1());
cout<<"Create a Second polynomial list "<<endl;
addlist.create(addlist.getstart2());
cout<<"\nFirst polynomial \n";
addlist.display(addlist.getstart1());
cout<<"\nSecond polynomial \n";
addlist.display(addlist.getstart2());
addlist.add();
cout<<"\nAddition of two polynomial \n";
addlist.display(addlist.getstart3());
return 0;
}
No comments:
Post a Comment