Here is a C++ program to sort list of names in ascending order and descending order.
C++ program to sort list of names in ascending order
#include<iostream>
#include<string.h>
using namespace std;
int main()
{
char *str[10],*temp;
int n,cmp;
cout<<"How many names to be entered : ";
cin>>n;
cout<<"Enter names : ";
for(int i=0;i<n;i++)
{
str[i]=new char[20];
cin>>str[i];
}
for(int i=0;i<n-1;i++)
{
for(int j=0;j<n-1;j++)
{
cmp=strcmp(str[j],str[j+1]);
if(cmp>0)
{
temp=str[j];
str[j]=str[j+1];
str[j+1]=temp;
}
}
}
cout<<endl<<"Sorted list : ";
for(int i=0;i<n;i++)
cout<<endl<<i+1<<".) "<<str[i];
return 0;
}

#include<string.h>
using namespace std;
int main()
{
char *str[10],*temp;
int n,cmp;
cout<<"How many names to be entered : ";
cin>>n;
cout<<"Enter names : ";
for(int i=0;i<n;i++)
{
str[i]=new char[20];
cin>>str[i];
}
for(int i=0;i<n-1;i++)
{
for(int j=0;j<n-1;j++)
{
cmp=strcmp(str[j],str[j+1]);
if(cmp>0)
{
temp=str[j];
str[j]=str[j+1];
str[j+1]=temp;
}
}
}
cout<<endl<<"Sorted list : ";
for(int i=0;i<n;i++)
cout<<endl<<i+1<<".) "<<str[i];
return 0;
}
OUTPUT
C++ program to sort list of names in descending order
#include<iostream>
#include<string.h>
using namespace std;
int main()
{
char *str[10],*temp;
int n,cmp;
cout<<"How many names to be entered : ";
cin>>n;
cout<<"Enter names : ";
for(int i=0;i<n;i++)
{
str[i]=new char[20];
cin>>str[i];
}
for(int i=0;i<n-1;i++)
{
for(int j=0;j<n-1;j++)
{
cmp=strcmp(str[j],str[j+1]);
if(cmp<0)
{
temp=str[j];
str[j]=str[j+1];
str[j+1]=temp;
}
}
}
cout<<endl<<"Sorted list : ";
for(int i=0;i<n;i++)
cout<<endl<<i+1<<".) "<<str[i];
return 0;
}
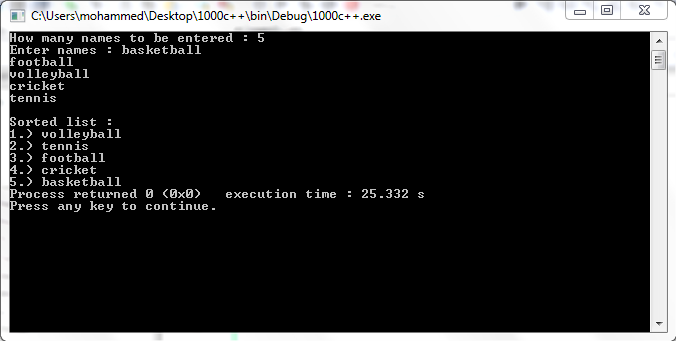
#include<string.h>
using namespace std;
int main()
{
char *str[10],*temp;
int n,cmp;
cout<<"How many names to be entered : ";
cin>>n;
cout<<"Enter names : ";
for(int i=0;i<n;i++)
{
str[i]=new char[20];
cin>>str[i];
}
for(int i=0;i<n-1;i++)
{
for(int j=0;j<n-1;j++)
{
cmp=strcmp(str[j],str[j+1]);
if(cmp<0)
{
temp=str[j];
str[j]=str[j+1];
str[j+1]=temp;
}
}
}
cout<<endl<<"Sorted list : ";
for(int i=0;i<n;i++)
cout<<endl<<i+1<<".) "<<str[i];
return 0;
}
No comments:
Post a Comment